Hi developers! Want to improve your .NET Core projects while keeping your code clean? You're in luck! Today, we'll explore MediatR and its Notification feature through a practical use case, showing how it can elevate your applications. Let's dive in!
What is MediatR
If you're new to MediatR, it's an open-source library that helps you implement the popular Mediator pattern in your .NET Core projects. MediatR acts as a central communication hub, allowing different parts of your application to talk to each other without direct dependencies. Say goodbye to tightly-coupled code and hello to cleaner, more maintainable projects.
MediatR Notifications
MediatR's Notification feature, also known as Publish, enables you to broadcast messages to multiple handlers asynchronously. This powerful mechanism informs your application's components about important events or changes without complicated logic or cumbersome wiring. It's like having your news network within your project!
Use Case:
Notify Email and SMS to Customers after Order Creation Event:
Imagine you're building an e-commerce application and need to notify customers via Email and SMS when their orders are created. Using MediatR Notifications, you can elegantly achieve this.
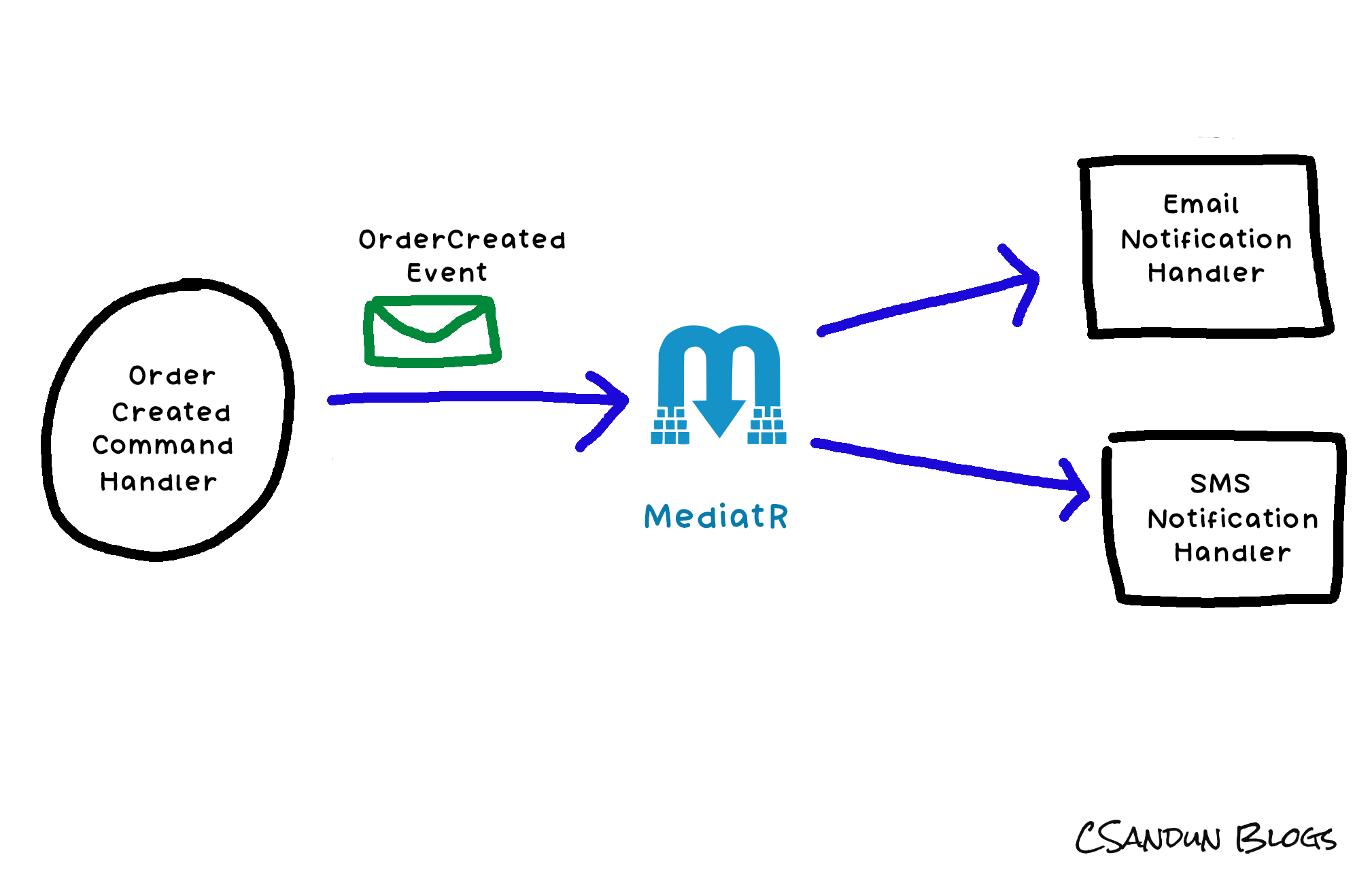
Explanation
In modern applications, executing tasks like sending emails or SMS notifications asynchronously ensures a smooth user experience and prevents unnecessary delays. For example, when creating an order, the primary focus should be completing the order process without depending on immediate responses from email or SMS services.
Leveraging MediatR Notifications allows efficient management of these asynchronous tasks, enabling the independent progression of the order creation process. This approach ensures application responsiveness and optimal performance while sending email and SMS notifications in the background without impacting the primary functionality of the order creation handler.
This is where out-of-process messaging comes in.
However, a single request can be handled by Multiple handlers. Some independent operation needs to occur after some events.
Practical
Explore my GitHub-linked codebase, where I've implemented the CQRS pattern using the MediatR library. Featuring a clean architecture within a single project structure, I've elegantly crafted an Order Create Command and corresponding Command Handler for the use case mentioned above.
1. First of all, Let’s create a notification and handler. It is also referred to as events. Add a class with the name `OrderCreatedNotification`.
public class OrderCreatedNotification : INotification
{
public Order Order { get; set; }
}
2. Now, I have created two handlers, one for sending Email and one for SMS.
public class OrderCreatedEmailHandler : INotificationHandler<OrderCreatedNotification>
{
private readonly ILogger<OrderCreatedEmailHandler> _logger;
public OrderCreatedEmailHandler(ILogger<OrderCreatedEmailHandler> logger)
{
_logger = logger;
}
public Task Handle(OrderCreatedNotification notification, CancellationToken cancellationToken)
{
// Send a confirmation email
_logger.LogInformation($"Confirmation email sent for order {notification.Order.Id}");
return Task.CompletedTask;
}
}
public class OrderCreatedSMSHandler : INotificationHandler<OrderCreatedNotification>
{
private readonly ILogger<OrderCreatedSMSHandler> _logger;
public OrderCreatedSMSHandler(ILogger<OrderCreatedSMSHandler> logger)
{
_logger = logger;
}
public Task Handle(OrderCreatedNotification notification, CancellationToken cancellationToken)
{
// Send a confirmation sms
_logger.LogInformation($"Confirmation sms sent for order {notification.Order.Id}");
return Task.CompletedTask;
}
}
You can elegantly incorporate the relevant logic within handlers, ensuring a clean and maintainable approach to implementing functionality in your application.
Trigger the Notification
Open `CreateOrderCommandHandler` and modify the handler method for publishing the order once saved in the database. Here I am representing a new order, including a new OrderId.
// Publish a notification that the order has been created
await _mediator.Publish(new OrderCreatedNotification() { Order = order }, cancellationToken);
The notification carries an OrderId
property containing the newly created order's ID. Using the _mediator.Publish()
method, the notification is asynchronously dispatched to all registered handlers interested in the OrderCreated
event.
Output
Swagger representation
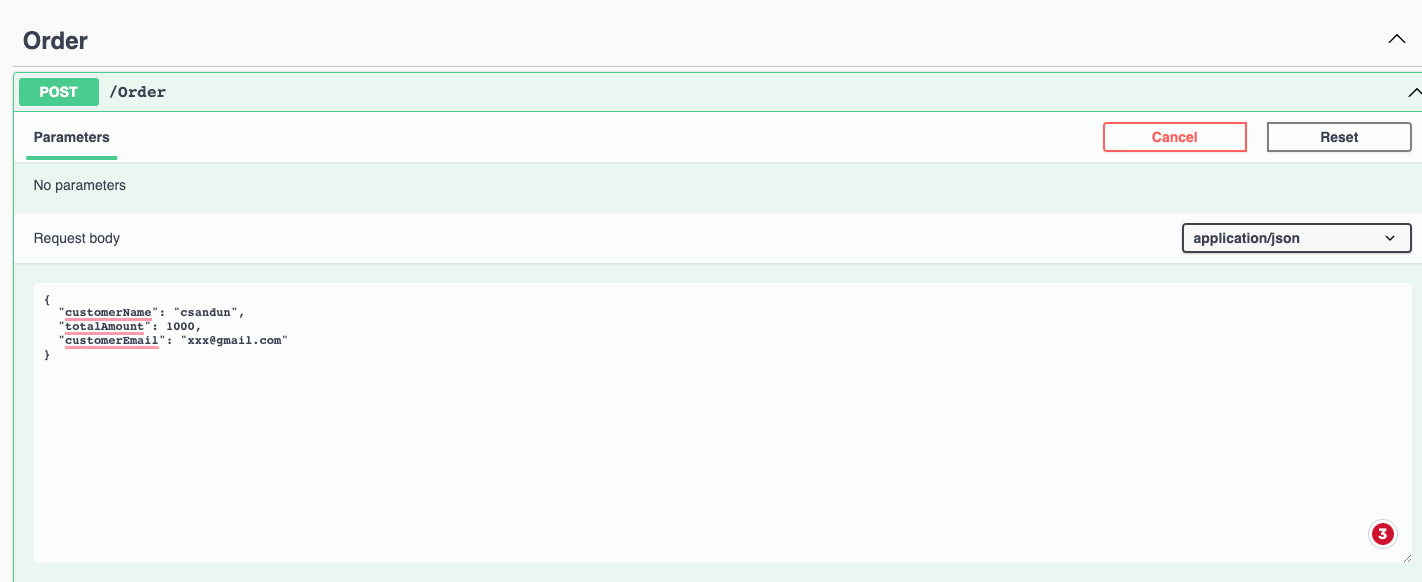
Output in terminal

Here we can see logs from both handlers.
Other use cases of MediatR Notifications
1.Event-driven architecture:
MediatR Notifications enable services in an event-driven system to communicate through events, promoting asynchronous reactions and reducing tight coupling.
2.Cross-cutting concerns:
Notifications handle concerns like logging, auditing, or monitoring by using separate handlers for each, ensuring clean separation and easy modification.
3.Integrating with external systems:
Notifications facilitate integration with external systems like messaging platforms (e.g., RabbitMQ, Kafka) or third-party APIs by processing notifications and sending messages or API requests as needed.
4.Caching and data synchronization:
Notifications maintain consistency and performance in distributed systems by alerting relevant services or components about data changes, triggering cache invalidation or data updates.
Let's discuss these concepts further in upcoming sections. Stay tuned for more insights on effectively incorporating this powerful tool in your .NET Core applications!
Conclusion
MediatR Notifications is a game-changer for your .NET Core projects in microservices architecture, streamlining communication and enhancing maintainability. Implementing this powerful feature can boost your application's capabilities while keeping your codebase clean and efficient.