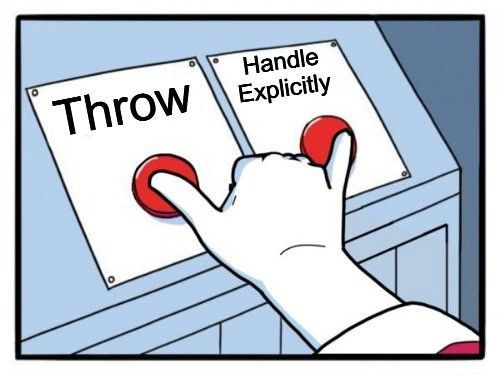
Do you still believe you should always throw domain exceptions from a .Net API? I followed many tutorials and blog posts, and they always encouraged us to throw exceptions from controllers and services. Here their point is the usage of the Custom Exception Middleware with the capability of global exception handling. Yes, it is correct; 👌 it helps to maintain a clean and reliable code base. We can handle all exceptions without defining an ugly huge try-catch block in every method.
Intro
But in some scenarios, you cannot always throw exceptions. So how can we handle exceptions explicitly?
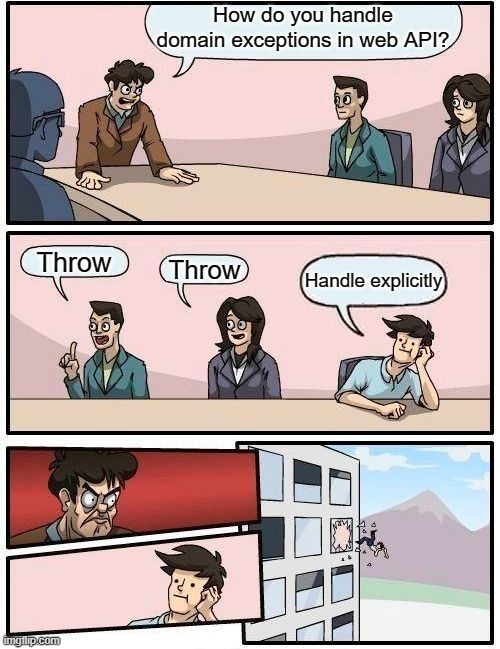
I want to build up a series of interconnected methods using one result dependent on another, like a flow operation. Some method wants to either throw exceptions or result from their method. But the main flow should not be disturbed and executed forward, and the error should be logged. The Plan is to store the above errors in one list. At the end of the process, I want to log all errors in a specific file. It seems to use an error-handling mechanism itself.
It is time to reveal how I accomplish this requirement using handle exceptions. It is explicit handling.
The ways I can handle exceptions in code
The old-school way adding an exception to the global list and returning a null value in the catch block. It is an awful solution when it comes to the domain-driven paradigm. You should always check null values at all places you use it. As well as unintentionally, you will meet NullPointExceptions through your code
Another way to use the combined result object. With returning value and exception together.
Much nicer. But I want more than this.
Apart from the above, I used a special class called Either<TLeft, TRight> Class.
Introducing Either<T1, T2>
Instead of returning Both with nullable properties, let's return Either with just one of them. When constructing an object, you can specify either a 'left' or a 'right' argument, but not both.
That's two types of parameters TLeft and TRight. You can then decide what to do if either situation occurs or return.
You can use Either class like this,
Using LeftOrDefault() or RightOrDefault()
You can specifically return the result object or an exception as a left or right value. Using LeftOrDefault() or RightOrDefault() you can get the returning value from this Either class.
Using match
A functional programming concept develops this Match method; you can call a function using the Match function according to your returning value type.
Enhancing for DoRight() action
Widely I used TRight value for returning exceptions. In some occurrences, I wanted to throw the returned exception immediately. Then I used DoRight() using action functionality in C# as below.
There is a simple, readable, no conditionals, no null checks, and no way to ignore the fact that exceptions may fail silently.
You can find the full code repository below.
Thoughts
You can use both Global Exception handling with throwing exceptions and handling explicitly. In handling exceptions, Either<TLeft, TRight> class is so clean and very nice in Domain-driven design exception handling.
See you again soon. ✌✌