Welcome to the "Byte-Sized" blog series
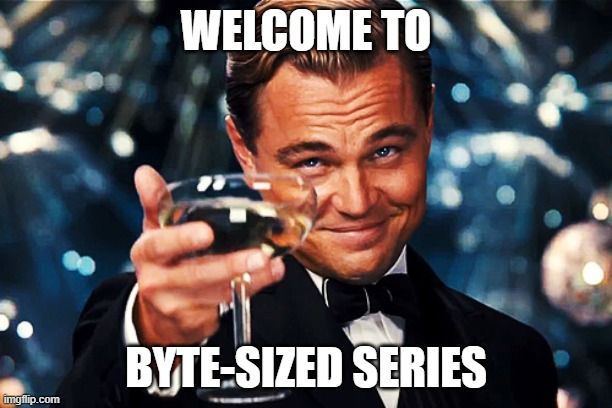
To kick off our series, let's apply Dependency Injection to Asp.net Core Console App.
Byte-Sized 01 Context
In my previous company, we relied heavily on console applications and windows services to automate various tasks and processes. In this context, I applied Dependency Inject into the Console Apps.
I got several benefits: increased flexibility and improved testability, better code reuse, easier maintenance, and improved code organization. Also, it helps to make the application more scalable and maintainable, ultimately resulting in better performance and quality.
How to apply Dependency Injection (DI) in a console application
1. Create dotnet 7 Console App
Create an empty console app using your IDE or dotnet CLI . Here I am using Rider. You can use blow command in dotnet CLI
dotnet new console
When create a console app, you can see a simple 'Hello world' application like this.
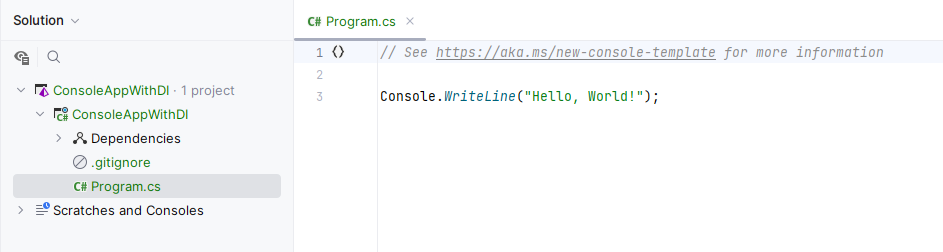
2. Create a mock service with interface as business logic
Well, Then I create a mock business service called CustomerService
. As well ICustomerService
. Today we are going to register this service in DI layer.
public interface ICustomerService
{
public void CalculateCustomerAge(int id);
}
public class CustomerService: ICustomerService
{
public void CalculateCustomerAge(int id)
{
Console.WriteLine("CustomerService:CalculateCustomerAge runs");
}
}
3. Setup Host
1. Add relevant NuGet packages
Add the Microsoft.Extensions.DependencyInjection
NuGet package to your project using dotnet CLI.
dotnet add package Microsoft.Extensions.DependencyInjection --version 7.0.0
Also we want Microsoft.Extensions.Hosting
to add hosting and startup infrastructure for the application
dotnet add package Microsoft.Extensions.Hosting --version 7.0.0
2. Put code snippets to create builder and DI layer
In the Main method of the console application, create a new HostBuilder
object, and register your concrete implementation with the service collection.
// create hosting object and DI layer
using IHost host = CreateHostBuilder(args).Build();
// create a service scope
using var scope = host.Services.CreateScope();
var services = scope.ServiceProvider;
// add entry point or call service methods
// more .....
// implementatinon of 'CreateHostBuilder' static method and create host object
IHostBuilder CreateHostBuilder(string[] strings)
{
return Host.CreateDefaultBuilder()
.ConfigureServices((_, services) =>
{
services.AddSingleton<ICustomerService, CustomerService>();
services.AddSingleton<App>();
});
}
Here you can register our CustomerService
mock service in Dependency layer.
4. Add Entry point to Application
Create a App
class as a entry class with Run()
entry method.
public class App
{
private readonly ICustomerService _customerService;
public App(ICustomerService customerService)
{
_customerService = customerService;
}
public void Run(string[] args)
{
_customerService.CalculateCustomerAge(1);
}
}
You can access console arguments from args
string property. Also you should register App
class in DI layer
IHostBuilder CreateHostBuilder(string[] strings)
{
return Host.CreateDefaultBuilder()
.ConfigureServices((_, services) =>
{
services.AddSingleton<ICustomerService, CustomerService>();
services.AddSingleton<App>(); // -> register App class
});
}
In Program.cs
we can call Run method as below.
// ....
var services = scope.ServiceProvider;
try
{
services.GetRequiredService<App>().Run(args);
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
//...
Time to run the your first "Hello World" Console application with Depencency Injection.
Full Program Class
using ConsoleAppWithDI;
using ConsoleAppWithDI.Services;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using IHost host = CreateHostBuilder(args).Build();
using var scope = host.Services.CreateScope();
var services = scope.ServiceProvider;
try
{
services.GetRequiredService<App>().Run(args);
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
IHostBuilder CreateHostBuilder(string[] strings)
{
return Host.CreateDefaultBuilder()
.ConfigureServices((_, services) =>
{
services.AddSingleton<ICustomerService, CustomerService>();
services.AddSingleton<App>();
});
}
Source Code repository
Resources
- Documentation
- From Tom Coory | From Youtube
Next Step:
The next step in this blog series can be to add an appsettings.json file and read configuration and add logs in your ASP.NET Core console application under DI framework. Keep in touch this valueable short series.